As an engineering leader, you’re always balancing speed with quality. But it’s easy to fall into the trap of writing convoluted code that works in the short term but leads to long-term maintenance headaches. Overcomplicated logic leads to lengthy testing cycles, harder bug fixes, and higher technical debt.
The complexity of your codebase increases as software projects do. As features are added a buildup of legacy code becomes hard to maintain. This can lead to significant challenges, including increased error rates, slower development cycles, and steep onboarding curves for new developers.
Yet many software engineering teams aren't aware that if applied correctly Cyclomatic Complexity is a key metric that can help you spot these pain points of code risks early. Understanding math is necessary but not sufficient for proper application.
In this article explore how Cyclomatic Complexity serves as a powerful metric to simplify code and reduce technical debt. By leveraging it effectively, you can pinpoint risky and overly complex areas in your codebase and transform them into clear, maintainable, and scalable solutions, ensuring higher quality and easier collaboration.
Whether you’re guiding a growing team or aiming to improve your existing codebase, follow this guide with practical steps to simplify your code, reduce bugs, and accelerate your development process.
What is Cyclomatic Complexity?
At its core, Cyclomatic Complexity (CC) is a software metric used to measure the complexity of a program by counting the number of linearly independent paths through its source code. In simpler terms, it helps you understand how many different execution paths your code could take during runtime.
Imagine you're navigating a maze. Each time you reach a point where the path splits into two or more directions, you have to make a decision about which way to go. The more splits and decision points there are in the maze, the more complex it is to navigate.
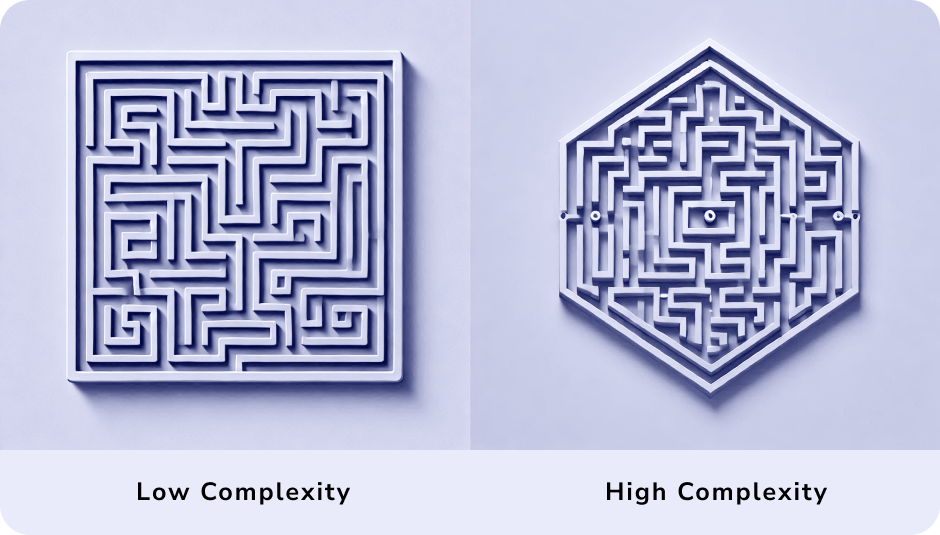
Developed by Thomas McCabe in 1976, this metric is calculated based on the control flow graph of a program. Each node represents a block of code (usually a statement or a group of statements), and edges represent the possible transitions between these blocks. The Cyclomatic Complexity score is determined by counting the number of decision points (such as if, for, and while statements) and calculating how many different paths can be taken through the code.
Cyclomatic Complexity Formula:
M=E−N+2P
Where:
- M = Cyclomatic Complexity
- E = The number of edges in the control flow graph
- N = The number of nodes in the control flow graph
- P = The number of connected components (usually 1 for a single program)
Why Cyclomatic Complexity Matters to Engineering Leaders?
By understanding Cyclomatic Complexity, engineering leaders can make informed decisions about code quality, team productivity, and long-term project sustainability. is crucial for maintaining high-quality software development. Here’s why:
- Code Maintainability: High Cyclomatic Complexity often signals that a function or module is difficult to understand and maintain. Code with high CC can become more prone to bugs and harder to refactor, which can slow down future development.
- Testability: The higher the Cyclomatic Complexity, the more potential paths exist through the code, which means more tests are needed to cover all possible scenarios. Lowering CC simplifies testing by reducing the number of paths, making it easier to achieve full test coverage.
- Bug Detection: High complexity increases the likelihood of defects. More branching and decisions in the code can lead to unexpected interactions and edge cases. By monitoring CC, you can catch high-risk areas early and improve overall code quality.
- Collaboration & Code Reviews: When multiple engineers collaborate on complex code over time, it becomes more difficult to comprehend and review. Reduced cyclomatic complexity makes code easier for engineers to understand various code functions, fostering a more collaborative environment.
- Improved Onboarding and Knowledge Transfer: Simpler and well-organized code can remarkably streamline the onboarding process, enabling new team members to quickly grasp and contribute to the project. With this practice, engineering leaders can easily facilitate smooth knowledge exchange among team members and keep the development team cohesive.
The Risks of High Cyclomatic Complexity
While some level of complexity is unavoidable, especially in large systems or highly dynamic applications, excessive complexity can lead to several problems:
- Increased Maintenance Overhead: Functions with high Cyclomatic Complexity are harder to update without inadvertently introducing bugs. They are also more prone to breaking when changes are made, as the number of possible interactions between components increases.
- Slower Debugging and Testing: Debugging and testing code with high CC can be extremely time-consuming. The more branches in your code, the more tests you need to run to ensure full coverage. This leads to increased testing time and, in some cases, delayed releases.
- Decreased Developer Productivity: Engineers may spend more time trying to understand and refactor complex code instead of adding new features or making improvements. This can reduce overall productivity and slow down project timelines.
- Difficult Code Reviews: High complexity makes code reviews harder. A reviewer may miss subtle bugs or fail to understand the full scope of the code’s functionality, leading to errors slipping through the cracks.
Interpreting Cyclomatic Complexity Scores
To interpret Cyclomatic Complexity, you can use these general guidelines:
- 1–10: Simple, easy-to-understand code with a low risk of bugs.
- 11–20: Slightly more complex, but still manageable. It might benefit from refactoring if the code grows.
- 21–50: Code is likely difficult to understand and maintain. Refactoring is recommended to simplify.
- 50+: Highly complex and at risk for significant issues. Major refactoring is necessary to reduce cyclomatic complexity.
How to Simplify Code and Reduce Cyclomatic Complexity?
Now that we understand why Cyclomatic Complexity is important, let’s look at practical strategies to reduce it and simplify your code.
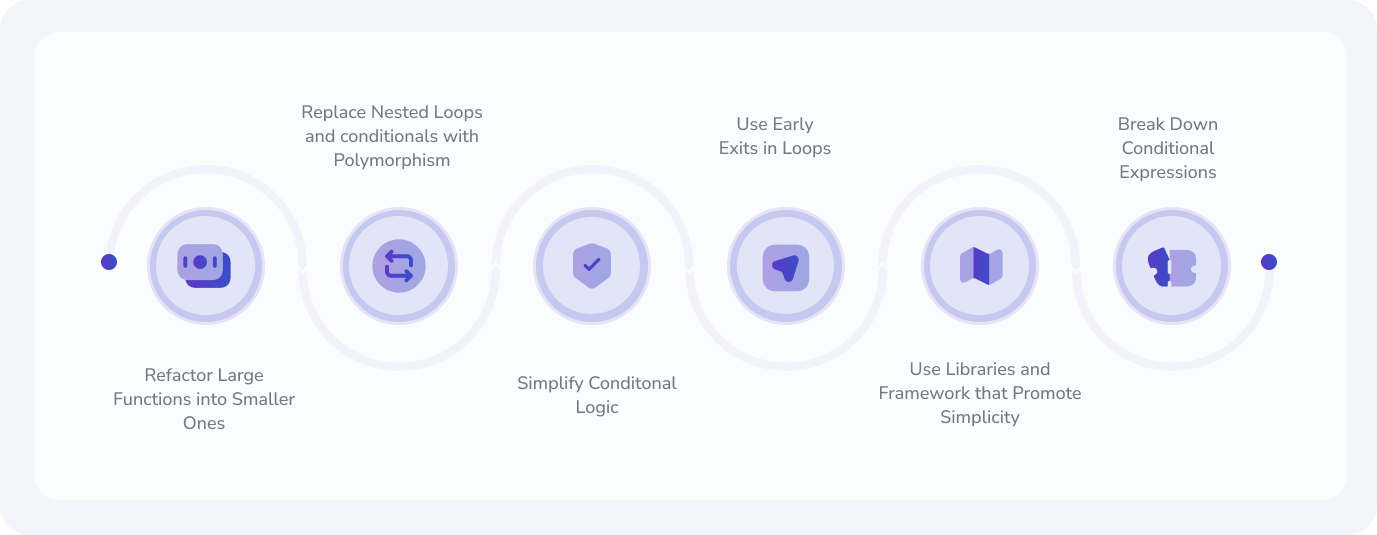
1. Refactor Large Functions into Smaller Ones
One of the best and most effective ways to reduce cyclomatic complexity is to break down large functions into smaller ones, which are also known as a Single Responsibility Principle (SRP). Refactoring reduces the decision points of functions, making code simpler and easier to maintain and test.
For example, rather than having one function doing input validation, data processing, and database tasks, you can refactor it into three separate focused functions to reduce cyclomatic complexity and improve code quality.
Benefits:
- Fewer branches in each function
- Improved testability and readability
- Easier debugging
2. Replace Nested Loops and Conditionals with Polymorphism
Nested loops and conditionals often contribute significantly to Cyclomatic Complexity. A simple way to reduce complexity is to replace these constructs with more modular solutions like polymorphism. In Object-Oriented Programming (OOP), polymorphism allows you to delegate behavior to different subclasses rather than using complex if or switch statements.
Instead of writing lengthy conditionals to check the type of an object or the state of the system, you could leverage polymorphic methods, where each class implements its own version of a shared interface. This keeps classes focused and also simplifies the logic for a single responsibility.
For instance, each payment method (such as Credit Card Payment, Pay Pal Payment, etc.) in a payment processing system might have its own class, each of which would implement the processPayment()method. Instead of writing a complex series of if-else conditions to handle different payment types, the appropriate class’s method is called directly.
Benefits:
- Reduced decision points
- Improved code reuse
- Better scalability and extensibility
3. Simplify Conditional Logic
Complex conditionals (e.g., deeply nested if, else if, or switch statements) often lead to high Cyclomatic Complexity because they introduce numerous decision points. Simplifying these conditionals is crucial for reducing complexity.
Consider the following strategies:
Early returns: Instead of deeply nested if statements, use early returns to handle edge cases or errors at the beginning of a function.
Before:
javascript
function processOrder(order) {
if (order.isValid) {
if (order.isPaid) {
// Process the order
}
}
}
After:
javascript
function processOrder(order) {
if (!order.isValid) return;
if (!order.isPaid) return;
// Process the order
}
Guard clauses: Use guard clauses to immediately handle conditions that would otherwise result in nesting. This makes the flow of the function clearer and reduces decision points.
Benefits:
- Fewer branches and decision points
- Improved readability
- Easier to reason about
4. Use Early Exits in Loops
Another common contributor to high Cyclomatic Complexity is complicated loop logic. Loops, particularly when combined with multiple conditions and deeply nested logic, can add unnecessary complexity. Instead of processing everything in a single loop, consider using early exits or breaking out of the loop as soon as the condition is met.
For example, consider this loop:
javascript
function findElement(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) {
// Do something
} else {
// Other logic
}
}
}
Instead of having an else branch for non-matching items, you could exit the loop once the target is found:
javascript
function findElement(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) {
// Do something
return;
}
}
}
This simplifies the logic and makes the flow of the function easier to follow.
Benefits:
- Reduces unnecessary iterations
- Simplifies control flow
- More intuitive and faster to read
5. Use Libraries and Frameworks that Promote Simplicity
While writing your own code to solve problems is often necessary, relying on well-established libraries and frameworks can drastically reduce complexity. Libraries typically come with well-tested, optimized methods that abstract away complicated logic.
For example, instead of writing custom algorithms for sorting or data validation, use existing JavaScript libraries like Lodash or Date-fns. These libraries provide reliable, high-performance utilities that are easy to integrate into your codebase, often with fewer lines and fewer decision points than if you wrote the logic from scratch.
Benefits:
- Reduced need for custom code
- More readable and maintainable code
- Better performance and reliability
6. Break Down Complex Conditional Expressions
Sometimes, Cyclomatic Complexity is increased by complex conditional expressions within a single statement. Simplifying these conditions or breaking them into multiple smaller, more understandable parts can make the code less complex.
For instance, consider the following:
javascript
if (user.isActive && user.role === 'admin' && (user.hasPermission || user.isSuperUser)) {
// Perform action
}
This could be broken down into more manageable, logically isolated conditions:
javascript
const isUserAdmin = user.role === 'admin';
const hasPermission = user.hasPermission || user.isSuperUser;
if (user.isActive && isUserAdmin && hasPermission) {
// Perform action
}
By making each condition a clear, separate variable, the logic becomes easier to follow and modify in the future while keeping Cyclomatic Complexity low.
Benefits:
- More readable code
- Easier to debug and extend
- Clearer intent in conditions
Tools for Measuring Cyclomatic Complexity
There are several tools available to help you measure Cyclomatic Complexity in your codebase. Here are a few popular ones:
- SonarQube: Offers detailed code quality reports, including complexity metrics and its an open source platform.
- ESLint: Analyzes JavaScript code for complexity and other issues.
- JDepend: Ideal for Java applications to measure complexity and related metrics.
- PMD: A static analysis tool that evaluates complexity for languages like Java and JavaScript.
When Not to Worry About Cyclomatic Complexity?
Cyclomatic Complexity is vital but not always definitive. Context is important because higher complexity may occasionally be required to achieve particular business objectives.
Here are a few exceptions to consider:
- Complex Business Logic: Sometimes, business requirements dictate that the code needs to be complex (e.g., for complex validation or financial calculations).
- Performance Optimisation: To maximise speed or memory use in some high-performance applications, more complicated code may be required.
- External Libraries: Using external libraries, You may not be able to control the complexity of the code, but this shouldn't deter you from focusing on making your own code simpler.
Conclusion
Cyclomatic Complexity offers a more refined way to measure code quality than traditional metrics like Lines of Code (LoC). It is a useful metric for ensuring your team delivers clean, maintainable code to control code complexity. By being aware of its implications, accurately calculating it, and adhering to best practices to keep it under control you can significantly reduce technical debt, improve code readability, benefit long-term team productivity and make sure that your software applications are scalable.
As an engineering leader, championing such a thoughtful approach in your software development lifecycle ensures a balance between innovation and quality is a must. By focusing on metrics that matter, you foster an environment where engineering teams thrive and deliver impactful, maintainable solutions.
Also, read: How Critical are DORA Metrics for Software Teams?
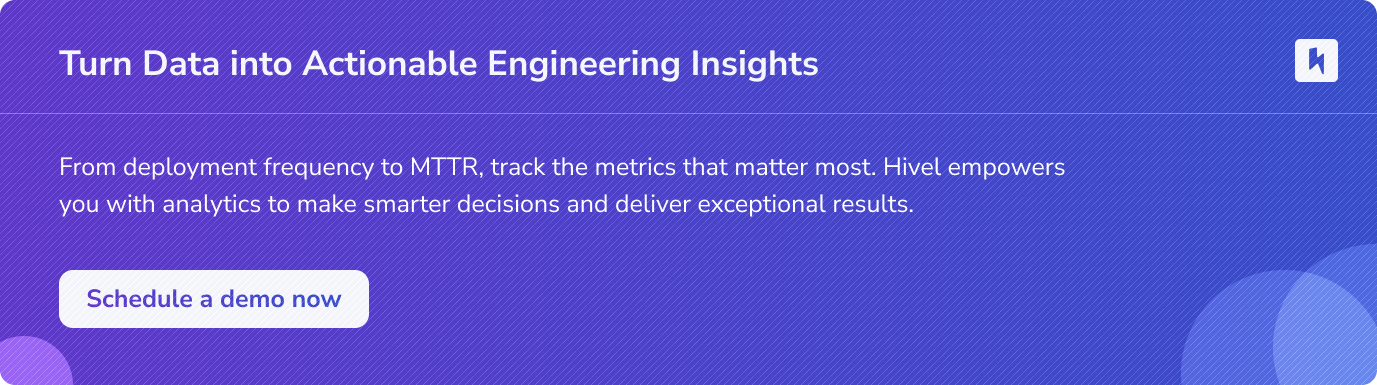